Your Guide Into Kotlin & Its Frameworks
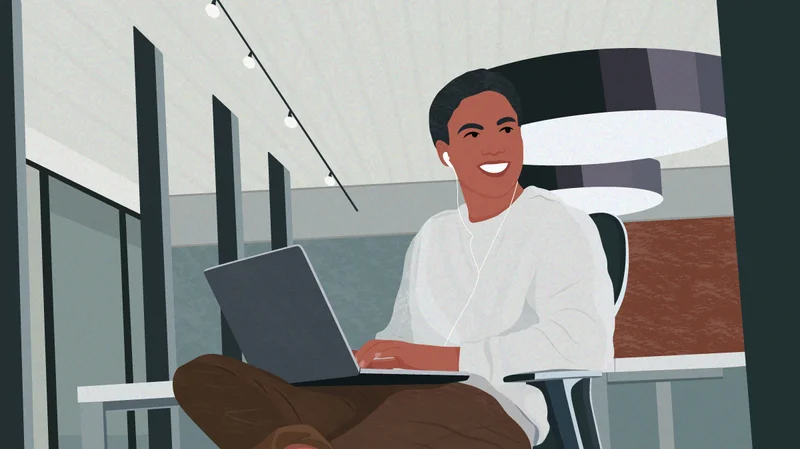
Introduction
We’ve been using Java for a long time here at Aristek Systems. Ten years ago we started with Java 7. Today, we’re working with Java 17.
All these years, the JVM ecosystem has been rapidly developing. New JVMs were released, like JRockit by Oracle and OpenJ9 by IBM. Also, new JVM languages came out, like Kotlin, Scala, Groovy, Clojure, and so on.
We found Java code to be pretty bulky and excessive. Also, new features are not very released very often. For example, Java Records was previewed in Java 14, but it was only fully released in Java 16.
So we looked for a JVM language that would suit us better. We gave Kotlin a try, and it didn’t disappoint. Kotlin turned out to be perfect for microservices.
This article is a brief overview of Kotlin and its frameworks. I’m writing it based on our experience with the language. We’ll discuss how Kotiln compares to Java; which frameworks should you try; and what does the actual code look like?
Kotlin
Kotlin was first released in 2011 by JetBrains. Yet the first officially stable version Kotlin 1.0 came out in early 2016.
In 2019, Google announced that Kotlin was the preferred language for Android apps.
With Kotlin you can write web apps, cross-platform mobile apps, and much more. For the most part, Kotlin is focused on JVM. But it also compiles to JavaScript or native code through LLVM.
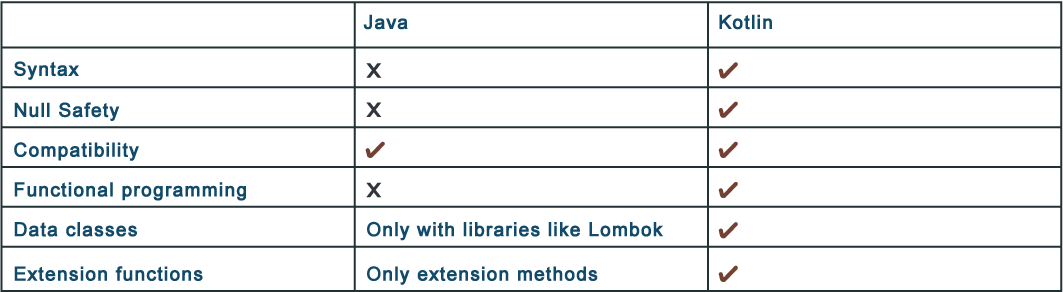
Java Vs. Kotlin
Kotlin Frameworks: Spring Boot & Ktor
If you want to build a web application in Kotlin, you’ll need to choose a framework: Spring Boot, Ktor, Micronaut, or something else.
One of the most popular Kotlin frameworks in 2023 are Spring Boot and Ktor. We’ve tried both, and here’s what we found.
Overall, Ktor is great for fast but smaller web applications. Spring Boot is perfect for more complex applications. But let’s learn more about them.
Spring Boot
Spring Boot is a very popular Java framework. Although this article is about Kotlin, Spring Boot also supports other languages, like Apache Groovy.
This framework is perfect for building web applications and microservices. It provides many features and functionalities, such as data access, security, and testing. Spring Boot has many libraries and tools that make it easy to build web applications.
Advantages Over Other Frameworks
- Huge Ecosystem. Spring Boot has many integrations with 3rd party services, databases, etc. That’s why you can do most daily tasks fast.
- Fast Development With Starters & Auto Configs. The annotation-based configuration makes it easy to set up and configure a Kotlin project. With Spring Boot you’ll write less boilerplate code.
- Support For Kotlin Features. Features like null safety and data classes reduce errors and make development much more pleasant.
- Powerful Testing Support. Things like the Spring Test framework make testing easy and efficient.
- Large Community With A Ton Of Educational Materials. You may easily find answers to most questions about Spring Boot.
Ktor
Ktor is another Kotlin framework for web applications. It’s written by JetBrains in Kotlin.
Advantages
- Very Little Overhead. Modular design allows to add and remove features as needed. It keeps your applications lightweight and efficient. With Ktor, your apps will take up fewer resources.
- Simple & Easy To Use API. This makes Ktor a great choice for novice developers.
- Suited For High-Performance Applications. Ktor supports asynchronous programming and coroutines.
Disadvantages
- Fewer Features Out Of The Box. Ktor has little overhead, but the flip side of it is that there are fewer overall.
- Smaller Community. Ktor is relatively new, so there are fewer resources and support compared to Spring Boot.
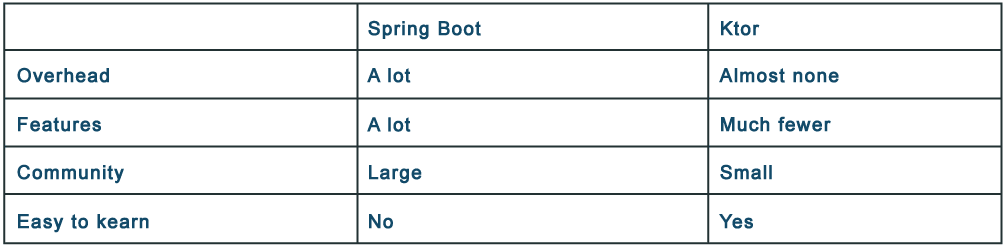
Spring Boot Vs. Ktor
Our Experience With Kotlin
Perhaps, the best way to try Kotlin in production is to write a microservice. If your application is built on microservice architecture, you can give Kotlin a try.
If you have a large monolithic application written in Java, you may want to use both Kotlin and Java simultaneously. In our experience, this will make your development quite messy, and bring out lots of surprises. So while it’s possible to use both languages together, we recommend sticking to one.
In our case, we were working on a project with microservice architecture. It had over 30 services on Spring Boot with Java.
We wrote one of the newer services in Kotlin with Spring Boot. After testing this approach in prod, we wrote a few more services this way. Next, we made a service on Kotlin with Ktor.
By now, we write all of our microservices in Kotlin. It is great for us.
Let’s take a look at some code examples comparing Java and Kotlin.
Code Examples
Collections
Optional findField(List fields, String fieldName) {
return fields.stream()
.filter(it -> it.getName().equals(fieldName))
.findFirst();
}
fun getField(fields: List, fieldName: String): Field? {
return fields.find { it.name.equals(fieldName) }
}
Object Creation
Factory getFactory() {
Factory factory = new Factory();
factory.setPrefetchCount(25);
factory.setDefaultRequeueRejected(false);
factory.setName("Factory");
return factory;
}
fun getFactory(): Factory {
return Factory().apply {
setPrefetchCount(10)
setDefaultRequeueRejected(false)
setName("Factory")
}
}
Conclusion
Today Kotlin is a mature language. Companies like Netflix, Tinder, and Airbnb already use it daily. Moreover, researchers in Data Science also use Kotlin.
With Kotlin, you can write fewer code lines. You can write code faster and with fewer errors. This makes it perfect for microservices. Kotlin is not limited to the JVM platform, so you can also use it in other ways.
There are lots of Kotlin frameworks, but Spring Boot and Ktor seem like the best options today. Spring Boot is better for larger applications, while Ktor is perfect for smaller ones.
All of this will make Kotlin more popular over the years. Hopefully, you’ll come to like the language as much as we do at Aristek Systems.