Four Handy JS APIs That You Didn’t Know About
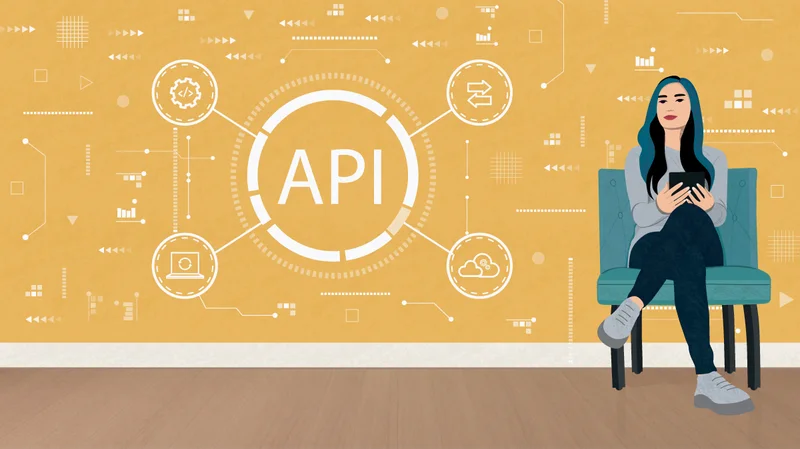
APIs are great, we all use some of them.
But there are many JavaScript APIs that few people heard of, yet are insanely powerful. Today, we’ll take a look at some of our favorite underdog APIs that we commonly use. Hopefully, you’ll come to like them as well.
Let’s see what they are all about and how you can use them.
Page Visibility API
When we wanted to check if the user has switched tabs or minimized a window, we used to stick with old-school solutions. Mostly, we used browser events like blur and focus.
But these solutions don’t work exactly the way we need. For example, blur fires whenever the page loses focus. This doesn’t always mean that the user has switched tabs. It can also happen when the user clicks on the search bar, a dialog box, the console, or an edge of the window.
This means that blur and focus can let us know if the page is active, but not if the user can read it.
Page Visibility API solves this problem. You can use this for this:
- When the user minimizes the page, you can pause videos, image carousels, or animations;
- If the page uses live data from an API, you can stop this when the page is minimized;
- Send user analytics.
How To Use It?
The API comes with 2 properties and an event. Here’s how you can access page visibility status with Page Visibility API:
- document.hidden
I love Page Visibility API, but I don’t recommend using this property, because it’s out of date. Instead, try document.visibilityState.
But if you’re still interested, this is a read-only property. It’s a boolean, so it can show if the document is visible in the background or not.
- document.visibilityState
I use this property instead of document.hidden. It does the same thing but adds more detail. Instead of the true or false responses, it shows 4 possible states of the page:- 1. Visible – it fires when the page is opened and active. With this state, the page is not minimized or hidden. The user can read the page.
- 2. Hidden – as the name says, the page is not active. It fires when the user switches tabs, but in Chrome it doesn’t fire when the user minimizes the page.
- 3. Prerender – this is the starting state of the page during the initial rendering. This status can change to another one, but none of the other statuses can switch back to prerender.
- 4. Unloaded – the page is being unloaded from the memory.
- visibilitychange
This event fires whenever document visibility changes. Very useful for analytics. The event can’t be canceled.
You can play around with the API here, although this simulation uses just the boolean document.hidden:
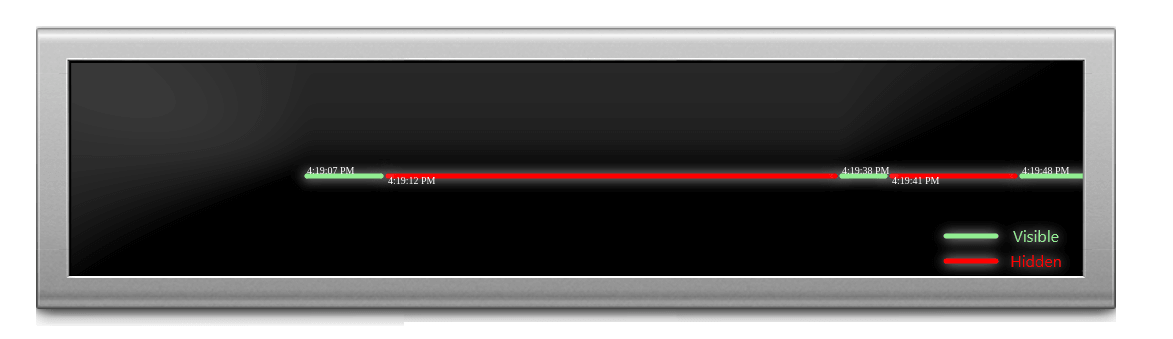
The Page Visibility API test by Web Performance Working Group
Browser Support
All modern browsers support Page Visibility API:
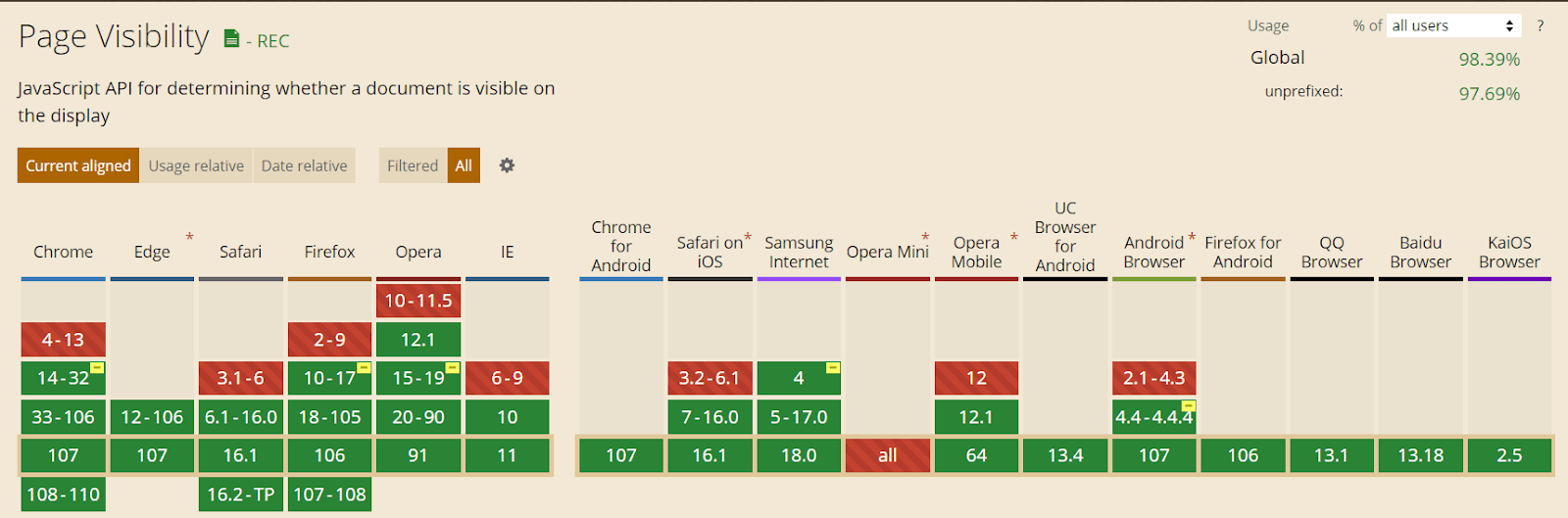
Page Visibility API Browser Support
WebShare API
WebShare API makes it easy for mobile users to share pages via a single button. Without the API, when we wanted to share a page via social networks, we added multiple icons:
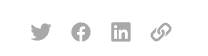
Icons Without WebShare API
With WebShare API you only need to add a single sharing button. This button will help with sharing via your installed apps:
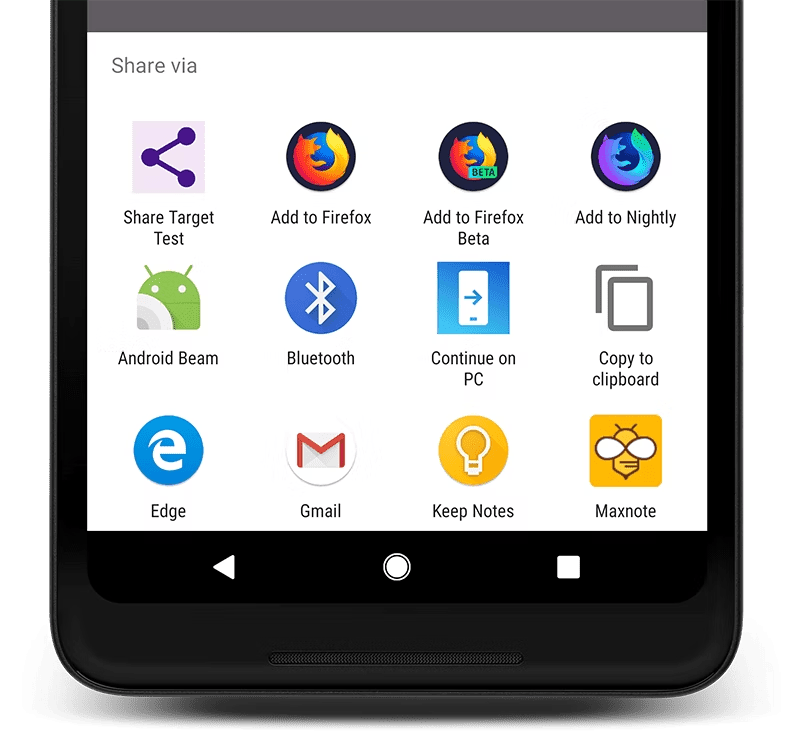
Sharing With WebShare API
Requirements
To secure the API, the developers included two limitations:
WebShare API can only be used on websites with HTTPS protocol. This way, the page is encrypted, and the websites won’t know which apps are installed on the user’s device. Moreover, to ease up the development, the API works on localhost.
The API can only work when it’s triggered by the user. It can’t fire during the initial page rendering or with setTimeout. Instead, a user needs to click the share button to fire the API.
Advantages
Without the API, a user can only share a page via the apps set up by the developer. With WebShare API, a user can share the page with any app that is installed on their device.
The page will load faster without the third-party scripts for individual services.
A developer doesn’t have to guess the social media apps to include for sharing. They can add a universal sharing button instead.
How To Use It?
The API works through the navigator.share method. Before using it, check if the browser supports WebShare API.
This is what it looks like in practice:
shareButton.addEventListener('click', event => {
if (navigator.share) {
navigator.share({
title: 'Check WebShare API',
url: 'https://example.com'
}).then(() => {
console.log('Api is running successfully!');
})
.catch(console.error);
} else {
// fallback
}
});
At this point, whenever a user clicks the Share button, the browser will pop up the sharing interface. Users can share content to any social network, email, SMS, or other apps that they have.
Browser Support
Note that some browsers don’t support WebShare API.
We want all users to still be able to share pages comfortably, so we have to build a backup solution as well. This could be a pop-up window with a few popular social network icons, like Twitter or Facebook.
The difference here is that, with the backup solution, sharing options are prewritten by developers. They may not include all the apps that the user has installed.
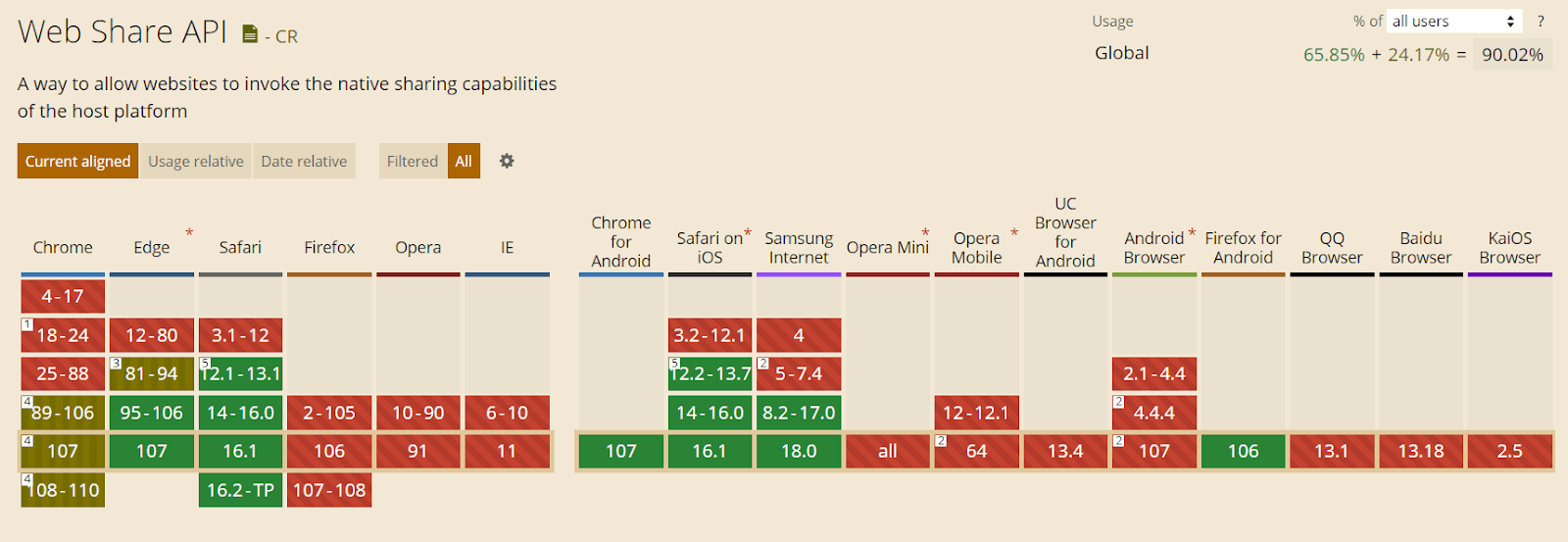
Webshare API Browser Support
Broadcast Channel API
Next is the Broadcast Channel API. With it, browser contexts like windows, tabs, frames, and iframes can communicate with each other.
With the API, when a user logs into a website, all other open tabs will also be logged in to the website.
For security reasons, the browser contexts must be of the same origin. That means, that they should share the same port, domain and protocol.
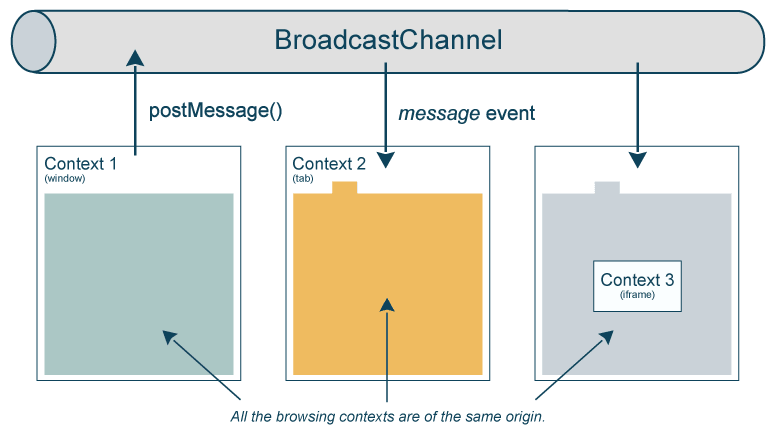
Broadcast Channel API
This is what Broadcast Channel API can do:
- Login a user across all open tabs;
- Download an asset in a single tab and reuse it in other open tabs. This can save traffic and make the pages open faster;
- Let a tab know that a process is finished in another tab;
- Launch a service worker to do a background job. This can sync data across multiple tabs.
- 2. Next, we’ll send a Hi Reddit message from the main page via.
Whenever you work with the API, you need to create a BroadcastChannel object first. It should have the class name that we want to share.</>
Now let’s send a message from the main pagebChannel.postMessage().
const bChannel = new BroadcastChannel("first_channel");
bChannel.postMessage('Hi Reddit!');
- 3. Finally, let’s receive this message on the login page.
Again, we start by creating a Broadcast channel object; then we receive the message through the bChannel.onmessage event.
const bChannel = new BroadcastChannel("first_channel");
bChannel.onmessage = function (event) { console.log(event); }
You can do this even if the browser pages are opened in different browser windows or if they are contained in frames.
Browser Support
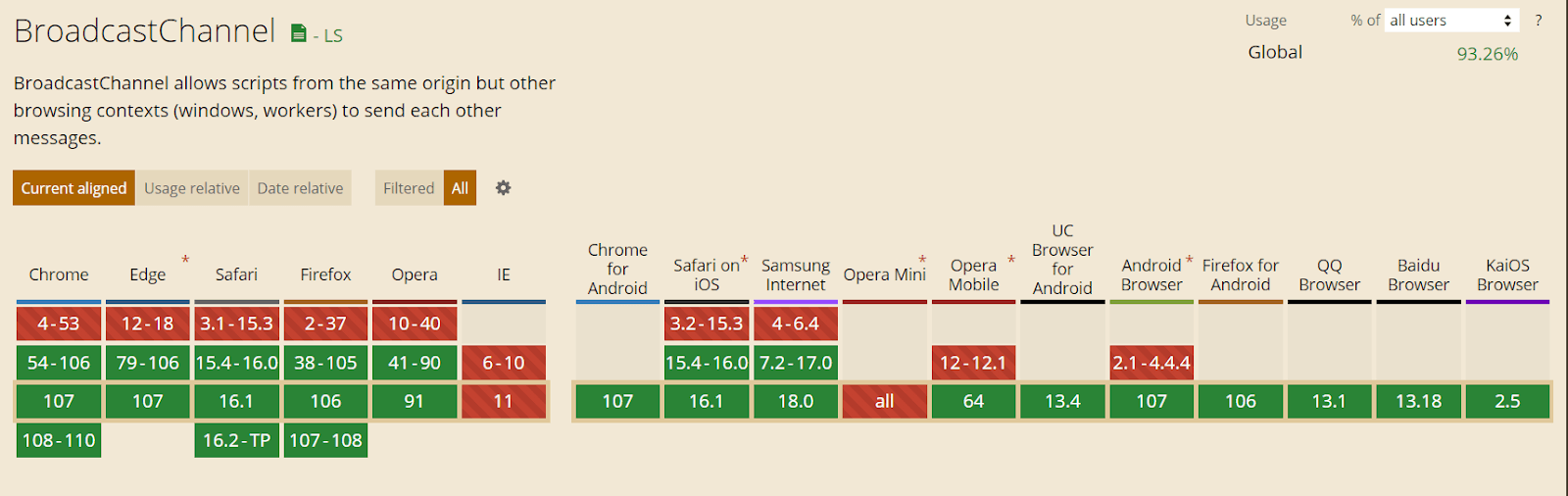
Broadcast Channel API Browser Support
Internationalization API
Some applications are localized to multiple languages. Yet, often a simple translation is not enough. Stuff like dates, measurement units, or numbers can be different across the world. If you don’t localize them, users aren’t going to be happy.
This is where Internationalization API (or I18n) comes in handy.
Historically, i18n was poorly supported in JavaScript. Developers used methods like toLocaleString() to localize their websites. But with this method, there’s no way to adjust data formats.
For example, you couldn’t add the weekday or exclude the year from the data format. And even if you didn’t need to tinker with such details, you couldn’t even convert the format to decimal from the percentile and so on.
How To Use It?
I18n API adds the Intl object. That object adds multiple constructors to work with language-sensitive data. Here are some of the most interesting constructors:
- Intl.DateTimeFormat() — to format dates and time;
const dateFormatter = new Intl.DateTimeFormat('en-US');
const day = dateFormatter.format( new Date('2022-11-14') );
// returns US format "11/14/2022"
- Intl.DisplayNames() — to format the names of languages, regions and scripts;
const regionNames = new Intl.DisplayNames(['fr'], {type: 'region'});
console.log(regionNames.of('FR'));
// France
- Intl.Locale() — to generate and manipulate locale identifiers;
const japanese = new Intl.Locale('ja-Jpan-JP-u-ca-japanese-hc-h12');
console.log(japanese.baseName);
// expected output: "ja-Jpan-JP"
console.log(japanese.hourCycle);
// expected output: "h24" "h12"
- Intl.NumberFormat() — to format numbers;
const amount = 1234.56;
const en = new Intl.NumberFormat("en").format(amount);
console.log(en);
// 1,234.56
- Intl.RelativeTimeFormat() — to formathttps://aristeksystems.com/portfolio/elearning-platform-for-k-12/ relative time, like “tomorrow”, “yesterday” and so on.
RelativeTimeFormat()
const rtf = new Intl.RelativeTimeFormat("es", { numeric: "auto" });
console.log(rtf.format(-1, "day")); // ayer
console.log(rtf.format(1, "day")); // mañana
Browser Support
Internationalization API is very adaptive, so most modern browsers support it.
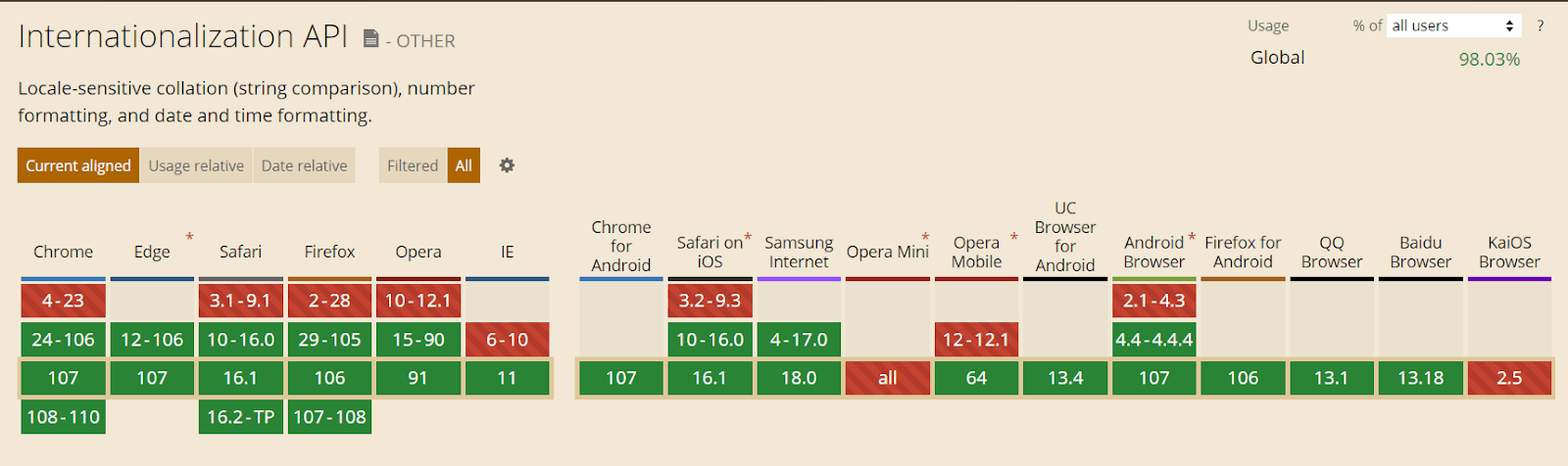
Internationalization API Browser Support
Conclusion
There are hundreds of Web APIs available to developers. It’s next to impossible to know all of them.
Even the least popular APIs can be very useful to an engineer. We’ve learned 4 of them today, but there are more to come.
I hope it’s been a good read, stay tuned.